Using Contentful with React: A Beginner's Guide
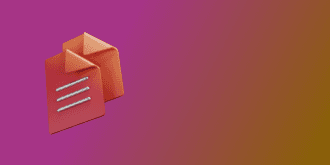
If you're looking to add a content management system (CMS) to your React application, Contentful is a great option to consider. It's a cloud-based headless CMS that allows you to easily manage and deliver your content to any platform or device. In this tutorial, we'll walk through the steps to set up Contentful and integrate it into a React application.
Before we get started, it's important to have a basic understanding of React and JavaScript. If you're new to these technologies, you may want to start with some online tutorials or resources to get up to speed.
Why Contentful is useful
Contentful is particularly useful for managing and delivering content in a React application because of its headless architecture. Because it's a headless CMS, it allows you to decouple your content management from your front-end presentation layer. This means that you can manage your content in one place and deliver it to multiple platforms or devices, without having to worry about how it's displayed.
Another advantage of using Contentful with React is that it provides a rich set of APIs and tools for managing and delivering your content. For example, you can use the Contentful Web App to create and edit your content, and the Contentful Management API to programmatically manage your content. You can also use the Content Delivery API to retrieve your content and use it in your React application.
One of the key benefits of using Contentful with React is that it allows you to manage your content in a structured and organized way. Contentful uses a concept called "content models" to define the structure of your content. This means that you can create templates for different types of content, such as blog posts, products, or events, and ensure that all of your content follows a consistent structure. This makes it easier to manage and organize your content, and it also makes it easier to retrieve and display it in your React application.
A headless CMS is a content management system that separates the backend content management functions from the frontend presentation layer. It allows you to manage your content in one place and deliver it to multiple platforms or devices, without being tied to a specific presentation layer or user interface.
Setting up Contentful
The first step in using Contentful with React is to set up a Contentful account and create a new space. If you don't already have an account, you can sign up for one at contentful.com.
Once you've created your account and logged in, click the "Create a new space" button to create a new space for your content. You'll be prompted to choose a name for your space and a default language. You can also choose to start with a sample space, which will give you some pre-populated content to work with.
After you've created your space, you'll need to create some content models. These are the templates for the types of content you'll be storing in your space. For example, you might have a content model for blog posts, another for products, and another for events. You can create content models by clicking the "Content models" button in the sidebar, and then clicking the "Create content model" button.
Installing the Contentful SDK
Once you've set up your space and created some content models, you'll need to install the Contentful SDK to your React application. To do this, you'll need to have Node.js and npm installed on your machine. If you don't have these already, you can download them from the Node.js website.
Once you have Node.js and npm installed, you can install the Contentful SDK by running the following command in your terminal:
npm install contentful
This will install the Contentful SDK and all of its dependencies to your project.
Connecting to Your Space
Now that you have the Contentful SDK installed, you can use it to connect to your space and retrieve content. To do this, you'll need to create a client object and pass it your space ID and access token. You can find these values in the "Settings" section of your space.
To create the client object, you can use the following code:
import { createClient } from 'contentful';
const client = createClient({
space: 'your-space-id',
accessToken: 'your-access-token'
});
With the client object created, you can now start retrieving content from your space.
Retrieving Content
To retrieve content from your space, you can use the getEntries
method on the client object. This method takes an options object as an argument, which allows you to specify the content you want to retrieve. For example, you can use the content_type
property to retrieve only entries of a certain content type, or the limit
property to limit the number of entries returned.
Here is an example of how you might use the getEntries
method to retrieve a list of blog posts:
client.getEntries({
content_type: 'blogPost',
order: '-sys.createdAt'
})
.then((response) => {
const posts = response.items;
// do something with the posts
})
.catch((error) => {
console.log(error);
});
This code will retrieve a list of blog posts ordered by their creation date, with the most recent post first. The then function will be called with the response object, which contains the list of posts in the items property.
Displaying Content in a React Component
Now that you know how to retrieve content from your Contentful space, you can display it in a React component. To do this, you'll need to make a request to the Contentful API and store the retrieved content in the component's state.
Here is an example of a React component that displays a list of blog posts:
import React, { useState, useEffect } from 'react';
import { createClient } from 'contentful';
const client = createClient({
space: 'your-space-id',
accessToken: 'your-access-token'
});
const BlogList = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
client.getEntries({
content_type: 'blogPost',
order: '-sys.createdAt'
})
.then((response) => {
setPosts(response.items);
})
.catch((error) => {
console.log(error);
});
}, []);
return (
<div>
{posts.map((post) => (
<div key={post.sys.id}>
<h2>{post.fields.title}</h2>
<p>{post.fields.body}</p>
</div>
))}
</div>
);
};
export default BlogList;
This component uses the useState
and useEffect
hooks to retrieve a list of blog posts from the Contentful API and store them in the component's state. The useEffect
hook is called when the component mounts, and it makes a request to the Contentful API to retrieve the posts. The then
function is called with the response object, which contains the list of posts in the items
property. The setPosts
function is called with the list of posts, which updates the component's state and triggers a re-render.
Finally, the component renders a list of posts using the map
function. Each post is wrapped in a div
element and rendered with its title and body.
Conclusion
In this tutorial, we've covered the basics of using Contentful with a React application. We've set up a Contentful account and space, installed the Contentful SDK, and used it to retrieve and display content in a React component.
While we've only scratched the surface of what's possible with Contentful and React, this should give you a good foundation for integrating a headless CMS into your application. With Contentful, you can easily manage and deliver your content to any platform or device, giving you the flexibility.